In Python, a string is basically an ordered sequence of characters to store text-based information. String data type in python supports lot of methods to process/manipulate the data.
In this article today, we will discuss about some of the basic operations which are supported by python string data type which are very useful to use and comes in handy while programming.
Before moving please have a look into Python String basics.
Length of string
In order to find length of string “len()” function is provided by python library which returns the length of string.
For eg:
len (‘test’) -> 4
Basic repetition and concatenation
Python library provides support of few operators which could be used by programmers on string data type.
- Operator “+” will concatenate strings.
- Eg: ‘abc’ + ‘xyz’ –> ‘abcxyz’
- Operator “*” will concatenate same string to multiplied number times.
- Eg: ‘abc’ * 3 –> ‘abcabcabc’
Indexing
In Python, strings content can be accessed by their position which is like other languages like C.
Characters in strings can be accessed by indexes based on the offsets which starts from 0 and ends at “length – 1”.
Characters can also be accessed by indexes using negative offsets which start from -1 (end of string) to beginning of it.
Let’s look into below diagram to understand indexing properly.
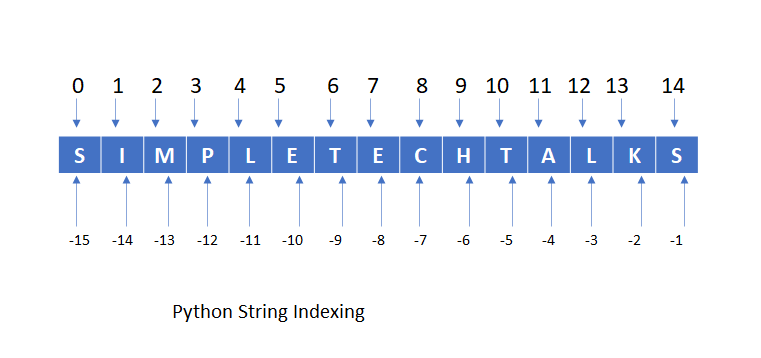
Properties of Indexing
- First character position is always at offset 0 whereas in case of negative offset, it would be -1.
- Offsets count from beginning to end whereas negative offset count from end to beginning.
- S[x] will fetch the character at location “x”.
- Eg: S[2] –> M, S[-3] –> L
Slicing of string
Python string data type supports accessing subsection of a string via index ranges. In this case, it will return a substring in one shot. Since, we are cutting the string in smaller pieces and hence this operation is called “slicing” in python.
Eg (Refer above image): S[1:3] –> ‘IM’, S[11:] –> ‘ALKS’
Properties of Slicing
- In case of S[i:j] –> index i is included, index j is excluded.
- Eg: S[1:3] –> ‘IM’
- S[1:] will fetch substring from index 1 to end of the string.
- S[:5] will fetch substring from index 0 to 5 where index 5 is excluded.
- S[:-1] will fetch substring from index 0 till last item where last item is excluded.
- [:] will fetch same string.
Extended Slicing
Python also supports an extended way to slice the string in which a step value could also be mentioned.
For eg: S[i:j:k].
In this case, a substring will be returned from index I to index j with step k. Please note that Step parameter is optional and if it is not mentioned then default value will be taken as 1.
Let’s look into a sample program to understand python string indexing and slicing.
S = 'SIMPLETECHTALKS'
S1 = 'Test!'
print ("Input String -", S)
print ("Length of String -", len(S)) # Checking Length of string
print ("String repeatation example - ", S1 * 3) # String repeatation example
print ('=' * 80) # String repeatation basic usage
print ('=' * 10, 'String indexing example', '=' * 10)
for index in range(0, len(S)):
print ('offset ', index, ' - ', S[index])
print ('=' * 10, 'String indexing example negative offset', '=' * 10)
for index in range((0 - len(S)), 0):
print ('negative offset ', index, ' - ', S[index])
print ('=' * 10, 'String Slicing example', '=' * 10)
print ("Slicing from 1 to 4 -", S[1:4])
print ("Slicing from 3 to end -", S[3:])
print ("Slicing from start to 10 -", S[:10])
print ("Slicing from start to end -", S[:])
print ("Slicing from -5 to -1 -", S[-5:-1])
print ("Slicing from start to -1 -", S[:-1])
print ('=' * 10, 'String Extended Slicing example', '=' * 10)
print ("Slicing from 1 to 10 with step 2 -", S[1:10:2])
print ("Slicing from start to end with step 2 -", S[::2])
print ("Slicing from 1 to 10 with step 2 -", S[-10:-1:2])
Let’s look into the output of above program.
Input String - SIMPLETECHTALKS
Length of String - 15
String repeatation example - Test!Test!Test!
================================================================================
========== String indexing example ==========
offset 0 - S
offset 1 - I
offset 2 - M
offset 3 - P
offset 4 - L
offset 5 - E
offset 6 - T
offset 7 - E
offset 8 - C
offset 9 - H
offset 10 - T
offset 11 - A
offset 12 - L
offset 13 - K
offset 14 - S
========== String indexing example negative offset ==========
negative offset -15 - S
negative offset -14 - I
negative offset -13 - M
negative offset -12 - P
negative offset -11 - L
negative offset -10 - E
negative offset -9 - T
negative offset -8 - E
negative offset -7 - C
negative offset -6 - H
negative offset -5 - T
negative offset -4 - A
negative offset -3 - L
negative offset -2 - K
negative offset -1 - S
========== String Slicing example ==========
Slicing from 1 to 4 - IMP
Slicing from 3 to end - PLETECHTALKS
Slicing from start to 10 - SIMPLETECH
Slicing from start to end - SIMPLETECHTALKS
Slicing from -5 to -1 - TALK
Slicing from start to -1 - SIMPLETECHTALK
========== String Extended Slicing example ==========
Slicing from 1 to 10 with step 2 - IPEEH
Slicing from start to end with step 2 - SMLTCTLS
Slicing from 1 to 10 with step 2 - EEHAK