Linked List is a data structure used for storing collection of data where successive elements are connected through pointers and the size of linked list can grow or shrink dynamically.
Linked List data structure is a type of Linear data structures.
In short, Linked list can be thought as similar to array in which traversal can happen through pointers instead of indexes.
Let’s have a look into some graphical examples of Linked List.
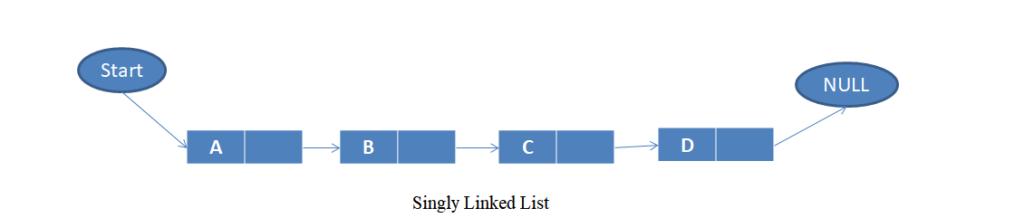
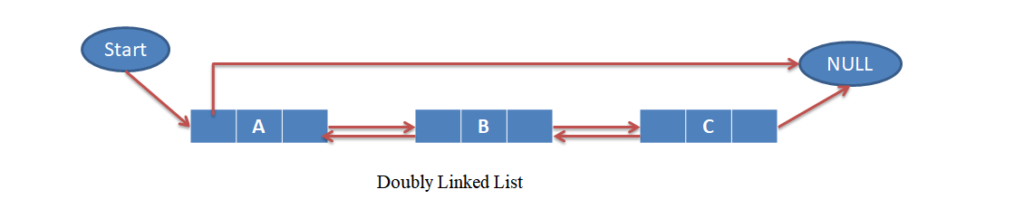
Properties of Linked List
- Last element points to NULL.
- Size can grow or shrunk dynamically.
- Memory can be allocated whenever it’s needed.
Let’s have a look into some of the most popular and useful topics in Linked List.
- Linked List basics and representation.
- Linked List basic implementation
- Linked List Operations: Insert
- Linked List Operations: Traverse and Search
- Linked List Operations: Delete
- Program to reverse a Linked List.
- Program to calculate length of a Linked List.
- Program to find Nth node from end of Linked List
- Program to detect and remove loop in Linked List.
- Program to swap two nodes in a Linked List.
- Program to reverse every K nodes in a Linked List.
- Program to find intersection of two Linked List.
- Program to check if Linked List is Palindrome.
- Adding two numbers represented by Linked List.